Fl_Native_File_Chooser
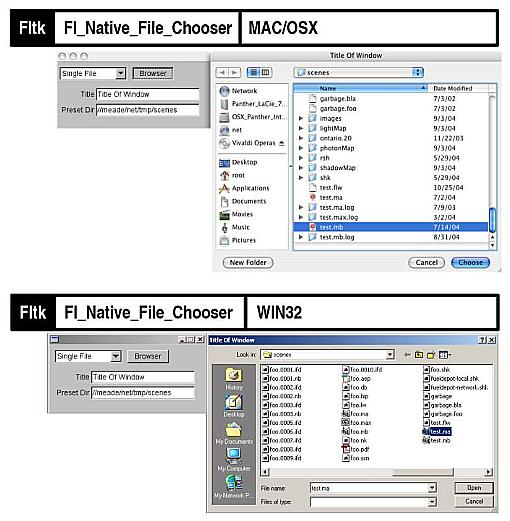
Examples of Fl_Native_File_Chooser on different platforms.
class Fl_Native_File_Chooser
Class Hierarchy
Fl_Native_File_Chooser
Include Files
#include "Fl_Native_File_Chooser.H"
Dowload source: Fl_Native_File_Chooser_src.zip
Description
This class lets an FLTK application easily and consistently access
the local operating system's native file chooser. Some operating systems
have very complex and specific file choosers that many users want access
to specifically, instead of FLTK's default file chooser(s).
Methods
The constructor for the Fl_Native_File_Chooser.
If the optional type is not specified,
BROWSE_FILE (browse to open a file) is assumed.
The type can also be set later with type().
The destructor; destroys any resources allocated to this widget.
int Fl_Native_File_Chooser::count() const
void Fl_Native_File_Chooser::directory(const char*)
const char* Fl_Native_File_Chooser::directory() const;
Sets the directory with which to start the chooser. If NULL is passed, or if no directory is specified, the chooser will attempt to use the last non-cancelled folder.
const char *Fl_Native_File_Chooser::errmsg() const
Returns a system dependent error message for the last
method that failed. This message should at least be flagged
to the user in a dialog box, or to some kind of error log.
const char *Fl_Native_File_Chooser::filename() const
const char *Fl_Native_File_Chooser::filename(int) const
const char *Fl_Native_File_Chooser::filter() const
void Fl_Native_File_Chooser::filter(const char*)
Gets or sets the filename filters used for browsing.
The default is "", which browses all files.
The format of each filter is a user description separated by the '\t' character from the wildcards.
On platforms that use the Fl_File_Chooser, description text within parentheses will be replaced by the wildcards, for compatibility with FLTK's API.
Individual filters are separated by newlines ('\n'). The final newline is optional.
Example: "Various web Files\t*.{htm,html,pl,cgi}\nText files (*.txt)\t*.txt"
The filter is ignored when browsing directories.
Examples of supported wildcard formats for 'filter'
are as follows, assuming the operating system's browser
supports wildcarding:
- "*" -- all files
- "*.txt" -- all files ending in .txt
- "*.{cxx,cpp}" -- all files ending in either .cxx or .cpp
- "*.[CH]" -- all files ending in .C or .H
void Fl_Native_File_Chooser::set_filter(int)
int Fl_Native_File_Chooser::set_filter()
The first form sets which filter will be initially selected.
The second returns which filter was chosen. This is only valid
if the chooser returns success.
The first filter is indexed as 0. If set_filter()==filters(),
then "All Files" was chosen. If set_filter() > filters(), then
a custom filter was set.
int Fl_Native_File_Chooser::filters()
Gets how many filters were available, not including "All Files"
void Fl_Native_File_Chooser::preset_file(const char*)
const char* Fl_Native_File_Chooser::preset_file()
Sets a default filename for the chooser. This may be used when
saving, and on most platforms can be used for opening files as well.
int Fl_Native_File_Chooser::show() const
Opens the current file chooser on the screen. This method
will block until the user has chosen something, or cancelled.
Return value:
- 0 - user picked a file, filename() will have the result
- 1 - user hit 'cancel'
- -1 - failed; errmsg() has reason
const char *Fl_Native_File_Chooser::title() const
void Fl_Native_File_Chooser::title(const char*)
Gets or sets the title of the file chooser's window.
The default title varies according to the platform, so you
are advised to set the title explicitly.
Fl_Native_File_Chooser::Type Fl_Native_File_Chooser::type() const
Fl_Native_File_Chooser::type(Fl_Native_File_Chooser::Type)
Gets or sets the current type of browser. Possible choices are:
Typical Platform Independent Usage
#include "Fl_Native_File_Chooser.H"
:
Fl_Native_File_Chooser *chooser = new Fl_Native_File_Chooser();
chooser->directory("/var/tmp");
chooser->title("Open a temporary file");
switch ( chooser->show() ) {
case -1: // ERROR
fprintf(stderr, "*** ERROR show() failed:%s\n", chooser->errmsg());
break;
case 1: // CANCEL
fprintf(stderr, "*** CANCEL\n");
break;
default: // USER PICKED A FILE
fprintf(stderr, "Filename was '%s'\n", chooser->filename());
break;
}